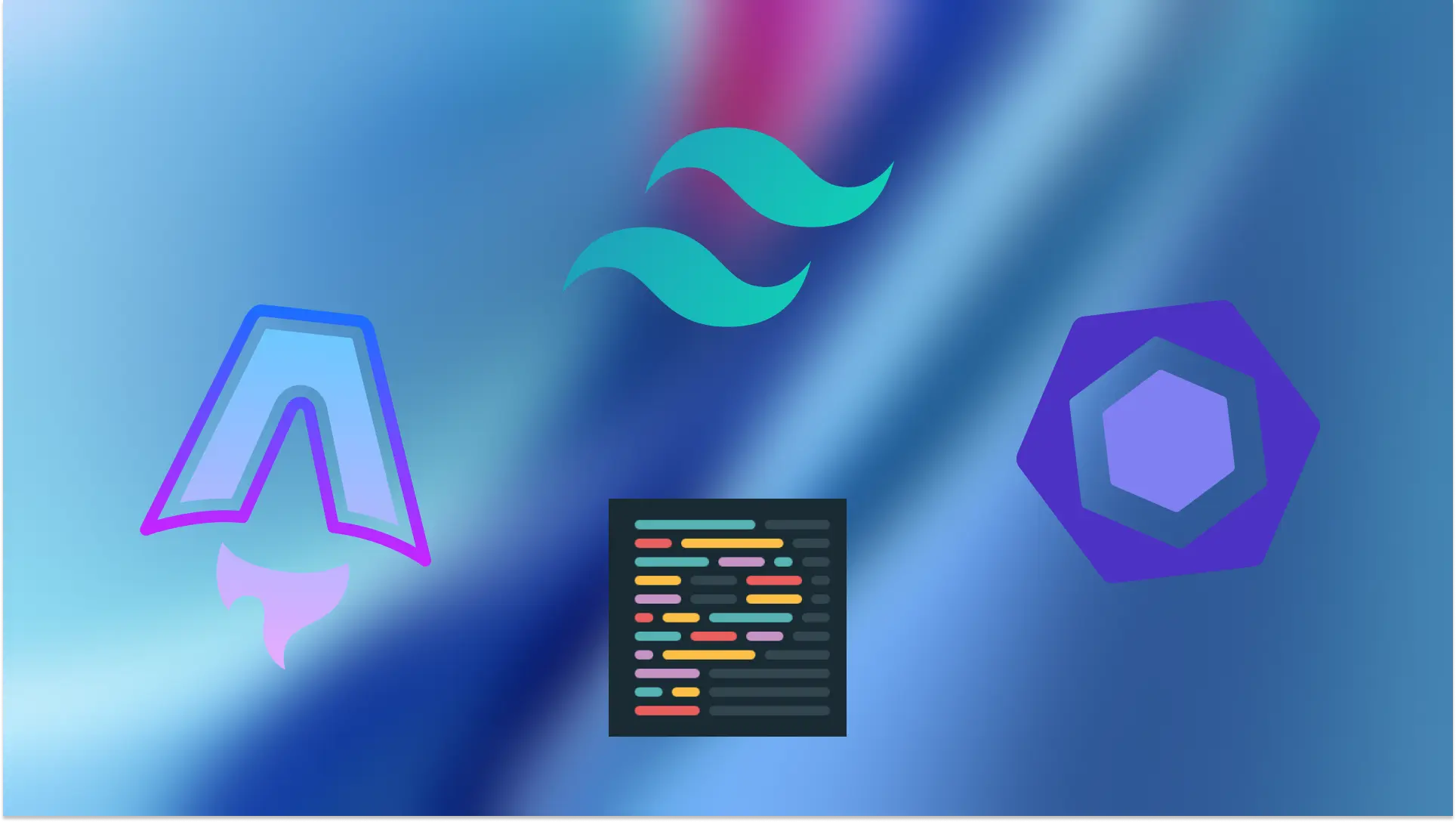
Beginner's Guide to Setting Up Astro: Astro + Prettier + ESLint + Tailwind CSS
Table of contents
- 0. Why?
- 1. Install and setup astro
- 2. Install and setup TailwindCSS
- 3. Test Tailwind CSS
- 4. Install and setup Prettier
- 5. Install and setup Eslint
- 6. Next Steps
0. Why?
By establishing a workflow with ESLint and Prettier, you can maintain code consistency, reduce potential errors, and improve readability in your Astro projects, ensuring a cleaner and more maintainable codebase. And Tailwind CSS is something that I use.
1. Install and setup astro
Navigate to the folder where you store your projects, like ~/Desktop, in your terminal and run the command.
npm create astro@latest
It will ask you to specify the location for your new project. For example, enter "./astro-tailwind" to create the "astro-tailwind" folder and set up Astro inside it. Open "astro-tailwind" with the following command.
code astro-tailwind
The above command only works when you have vs code installed.
Once opened in the code editor, you'll find files and folders in your project. To open the terminal, use Ctrl + `. Then, run the following command to start your development server. Astro provides some UI by default. You should see it once your server is up and running. I'll share a screen capture for reference and this UI may change in future.
npm run dev
Visit your localhost, and access the astro pre populated dashboard. It should show something like this.
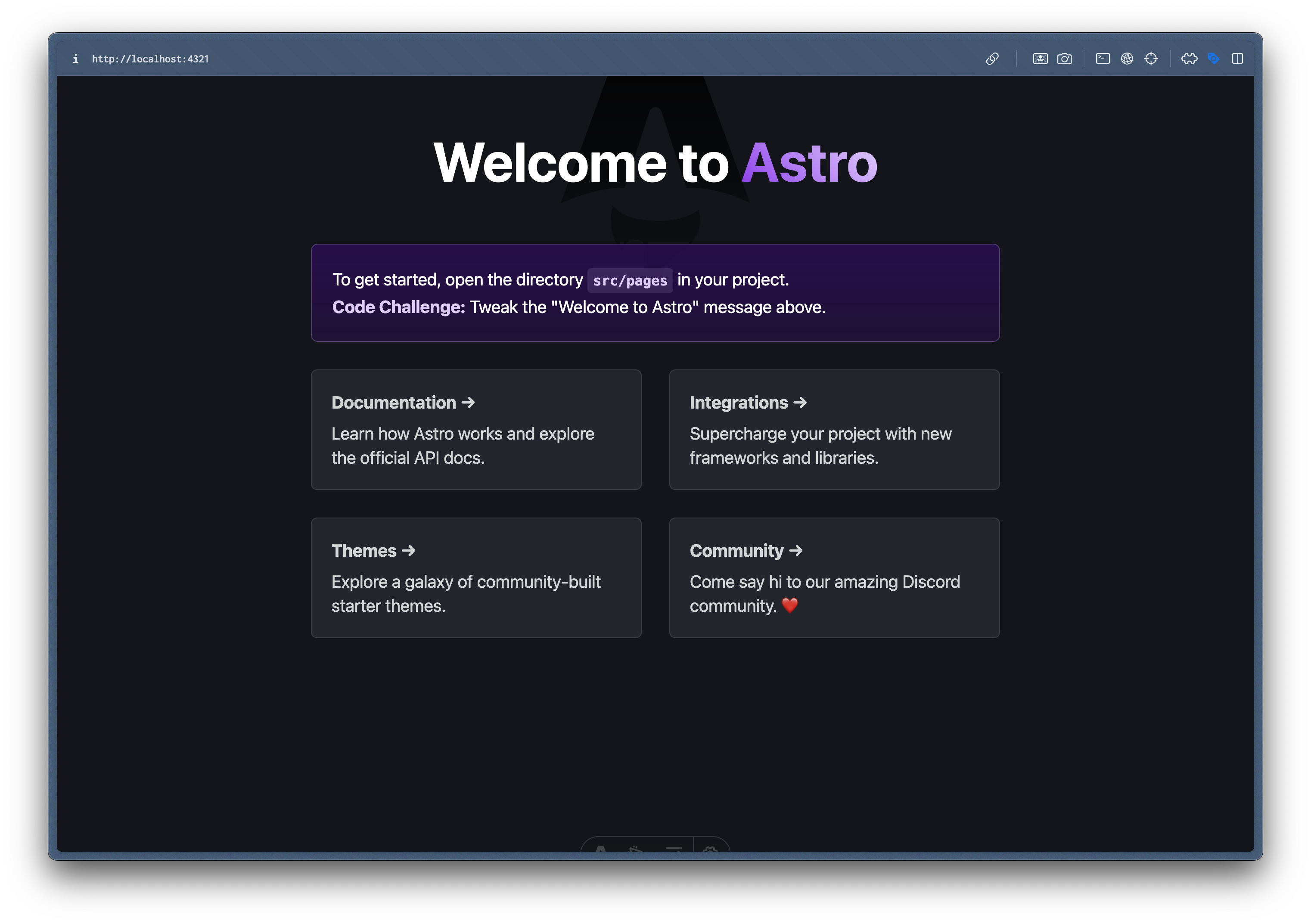
Next install astro extension on your VS Code
2. Install and setup TailwindCSS
If your terminal is running local server, exit using Ctrl + C, then run the following command to install tailwind.
npx astro add tailwind
This will do 2 things.
- Creates a tailwind.config.mjs file in your project's root directory.
- Integrates tailwind() in astro.config.mjs file.
That's it. Manual installation and configuration are unnecessary. Refer to this documentation if interested.
Bonus tip: Install Tailwind CSS Intellisense on your VS Code before you proceed.
3. Test Tailwind CSS
Now let's cleanup your index.astro file from your src/pages folder. Navigate to src/pages/index.astro and remove everything except Layout wrapper and add h1 tag with tailwind classes. Your index.astro file should now look something like this.
---
import Layout from '../layouts/Layout.astro';
---
<Layout title="Welcome to Astro.">
<h1 class="text-white text-3xl p-10">Hello World ;)</h1>
</Layout>
To verify this, restart your development server by running this command in your terminal.
npm run dev
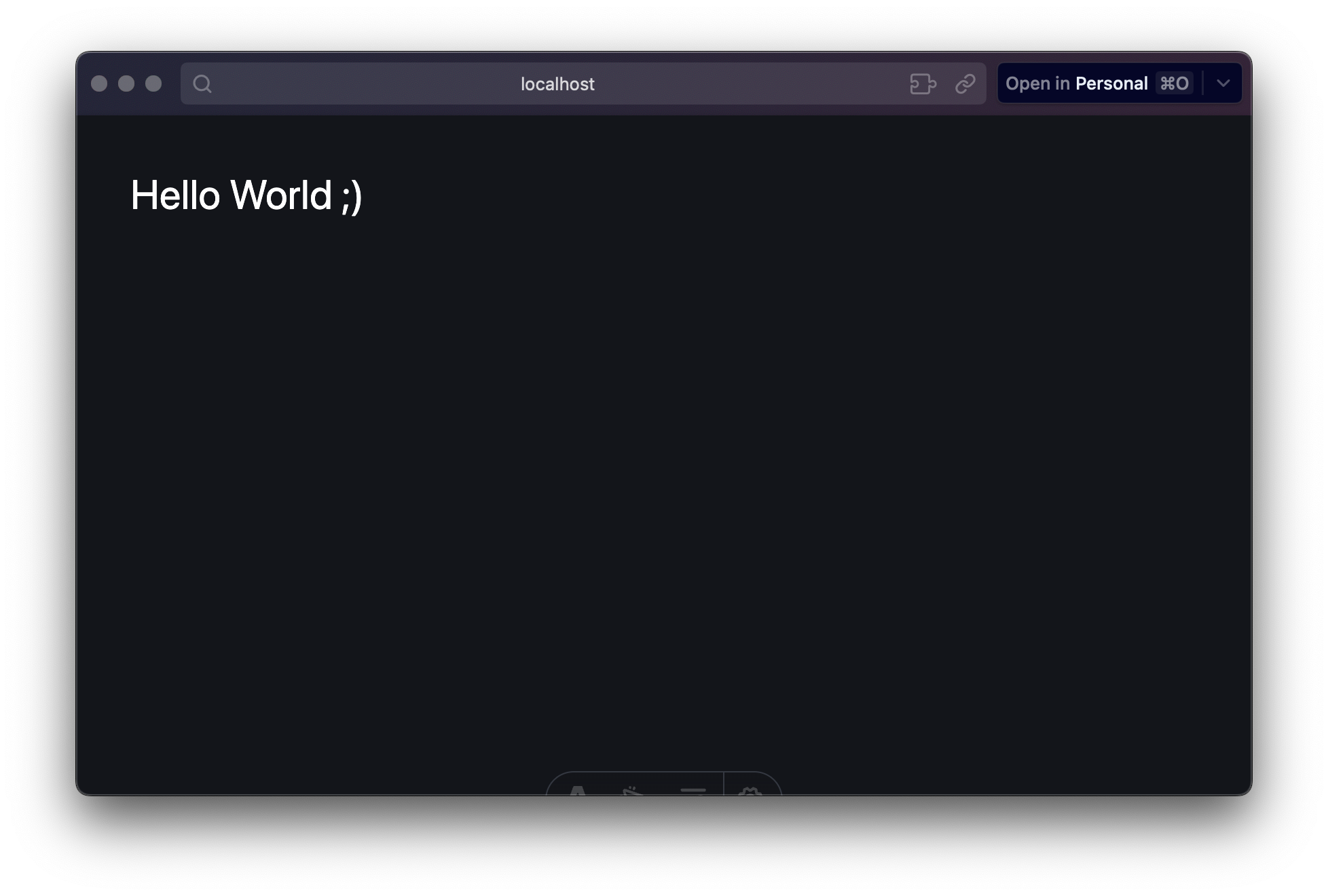
Congratulations on successfully setting up Tailwind with Astro if you see a similar output. In the image above, you can observe that padding, text color, and size were applied as specified in the index.astro file for our h1 tag.
4. Install and setup Prettier
Install the prettier extension on VS Code, and use the following command to install prettier packages
npm install --save-dev prettier prettier-plugin-astro prettier-plugin-tailwindcss
If you haven't installed tailwind css, you can skip adding prettier-plugin-tailwindcss. After installing Prettier, Generate .prettierrc.json and .prettierignore files in your project's root folder based. And feel free to explore official configuration. If you're using mac, you can use touch command as follows.
touch .prettierrc.json && touch .prettierignore
You can refer to prettier official docs to set it up according to your preferences. Alternatively, use the provided code in ~/.prettierrc.json.
{
"trailingComma": "none",
"overrides": [
{
"files": "*.svg",
"options": {
"parser": "html"
}
},
{
"files": "*.astro",
"options": {
"parser": "astro"
}
}
],
"plugins": ["prettier-plugin-astro", "prettier-plugin-tailwindcss"]
}
And navigate to ~/.prettierignore and insert below code.
dist
node_modules
Enable Prettier for Astro files by following these steps:
1. Create a settings.json file in .vscode folder
2. Add following code in it.
// ~/.vscode/settings.json
{
"prettier.documentSelectors": ["**/*.astro"],
"editor.defaultFormatter": "esbenp.prettier-vscode"
}
5. Install and setup Eslint
Use this command and install following packages.
npm i --save-dev eslint eslint-plugin-astro @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-plugin-jsx-a11y
If you're not using TypeScript, skip installing @typescript-eslint/parser and @typescript-eslint/eslint-plugin packages. Create a new file named .eslintrc.cjs in your project's root folder.
touch .eslintrc.cjs
copy paste the following code in it.
module.exports = {
extends: [
"plugin:astro/recommended",
"plugin:jsx-a11y/strict",
"eslint:recommended",
"plugin:@typescript-eslint/recommended"
],
overrides: [
{
files: ["*.astro"],
parser: "astro-eslint-parser",
parserOptions: {
ecmaVersion: "latest",
parser: "@typescript-eslint/parser",
extraFileExtensions: [".astro"],
project: "./tsconfig.json"
},
rules: {}
}
],
env: {
node: true
},
rules: {
"@typescript-eslint/ban-ts-comment": "warn"
}
};
Update your ~/.vscode/settings.json with this configuration.
// ~/.vscode/settings.json
{
"files.autoSave": "onFocusChange",
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
"editor.codeActionsOnSave": {
"source.organizeImports": "explicit"
},
"eslint.validate": [
"javascript",
"javascriptreact",
"astro", // Enable .astro
"typescript", // Enable .ts
"typescriptreact" // Enable .tsx
],
"prettier.documentSelectors": ["**/*.svg", "**/*.astro"],
}
That's it. ESLint should be working like a charm.
6. Next Steps
I've set up editorconfig and lefthook in my project. Explore these for additional functionalities.